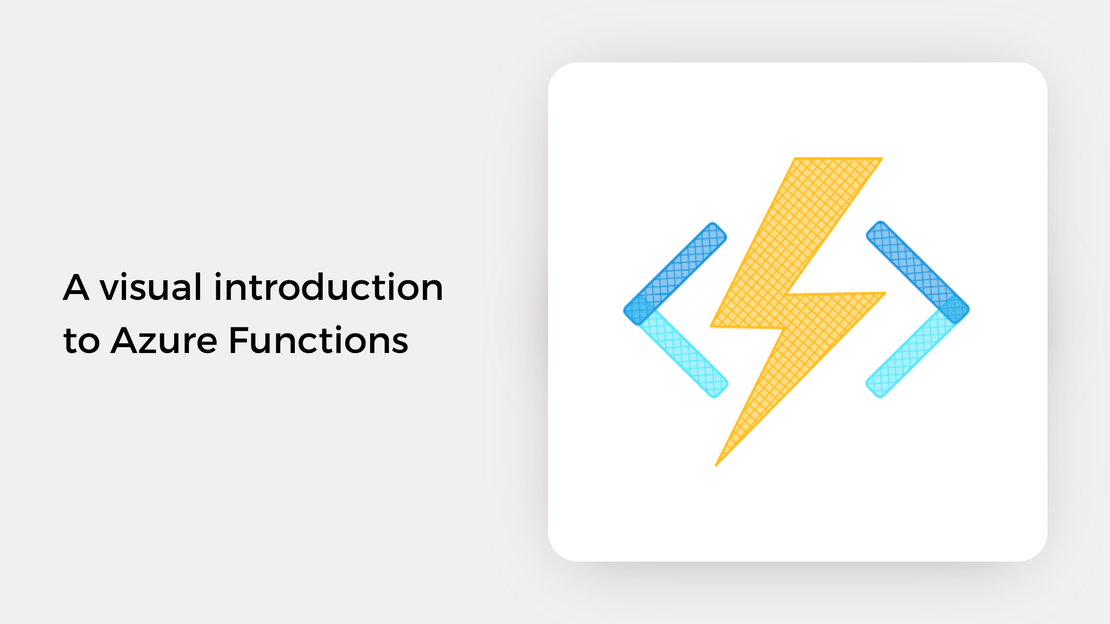
A visual introduction to Azure Functions
Explore the key concepts and use cases of Azure Functions with an illustrated guide and set up your local development environment.
In the previous post, we covered the fundamental concepts and common use cases of Azure Functions, as well as how to set up the necessary tools for developing Azure Functions. In this post, we will explore triggers and bindings, two core components of Azure Functions that specify how a function is run and interacts with services to send and receive data. I will also provide examples in Python using the v2 programming model.
In this article, you will:
The main points of this article are summarized in the following illustrated guide. It provides an overview of Azure Functions triggers and bindings and examples of how to define them in Python.
A function doesn’t run continuously but instead it executes in response to events through triggers. A trigger defines how a function is invoked. For example, a function can:
In Azure Functions, each function must have exactly one trigger. If you need to handle different types of events, you should create multiple functions with the same logic. Like passing arguments to a method, a trigger can also pass data to the function, such as the request body in a POST HTTP request.
functions.json
. The core definition is similar.In the Python v2 programming model, you define the trigger for a function directly in the code using a decorator. For example, to execute a function on a schedule, you can use the timer_trigger
decorator and specify the cron schedule along with the name of the variable that represents the timer object in the function code.
|
|
Another example is running a function when a blob is created or updated in Azure Blob Storage. You can use the blob_trigger
decorator and specify the following parameters:
inputblob
, which will hold the content of the blob in the function code.path
property to define variables or include filtering criteria that specify which blobs trigger the function. In the example below, the name
variable references the name of the blob, and the function triggers only on .png
files.
|
|
Azure Blob Storage also supports Python SDK-type bindings, which let you directly access the underlying blob and container client. In that case, the arg_name
property references a client object.
Another important component of Azure Functions is bindings. Bindings are used to connect Azure Functions with other Azure services. A binding can be used to connect to either a data source (input binding) or a data destination (output binding). An Azure service may offer both input and output bindings. The difference between an input and an output binding lies in the direction of data flow: an input binding passes data to a function, while an output binding transfers data from a function to another service. While every function must have exactly one trigger, it can have multiple input and output bindings.
Azure Blob Storage, Azure Cosmos DB, and Azure SQL are a few examples of input bindings. Examples of output bindings include Azure Blob Storage, Azure Service Bus, Azure Cosmos DB, and HTTP (for responding to an HTTP request).
By abstracting connection logic, bindings simplify integration with Azure services and eliminate the need to write service connection logic. Azure Client SDKs can always be used to connect to services in the case that the necessary binding is not available.
In the Python v2 programming model, you define input and output bindings directly in the function code using decorators, similar to triggers. For example, you can use the blob_output
decorator to upload a file to Azure Blob Storage.
In the example below, the set
method of the outputblob
object is used to write data to Blob Storage. The underlying data type is specified in the data_type
property, which in this case is bytes
.
|
|
If you are interested in learning more about triggers and bindings in Azure Functions, you can review the following resources:
Explore the key concepts and use cases of Azure Functions with an illustrated guide and set up your local development environment.
In this article, you will deploy a function project to Azure using Visual Studio Code to create a serverless HTTP API.
In this article, you will create a Python Azure Function with HTTP trigger to consume a TensorFlow machine learning model.
Explore how to automate the build and deployment process for a visual novel game using GitHub Actions and Azure Static Web Apps.