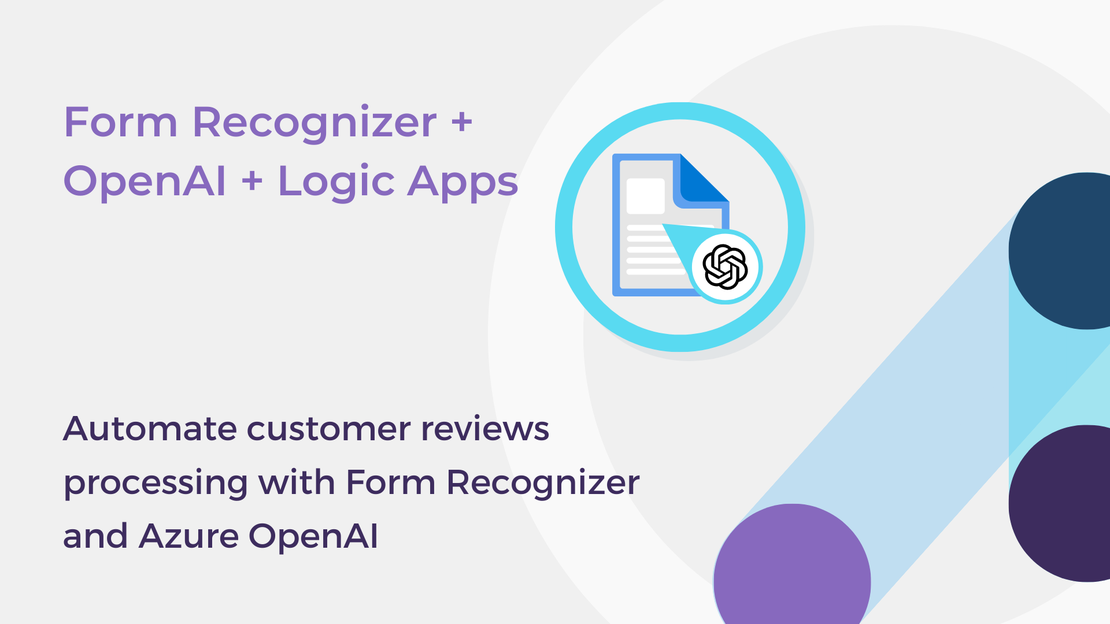
Automate customer reviews processing with Form Recognizer and Azure OpenAI
In this article, you will build a document processing solution using Form Recognizer, Logic Apps, and Azure OpenAI.
Azure Form Recognizer is an Azure Applied AI service that lets you easily analyze documents and extract text fields, data and tables and identify key-value pairs. In this article, you will learn how to extract common fields from sales receipts using the Azure Form Recognizer client library for Python.
You will learn how to:
To complete the exercise, you will need to install:
You will also need an Azure subscription. If you don’t have one, you can sign up for an Azure free account.
Form Recognizer is one of Azure’s cloud-based artificial intelligence services that can be used to build applications for automatic document analysis and information extraction. Form Recognizer uses deep learning models to analyze document data, extract text and values, and map relationships between data fields. Using the Form Recognizer API, you can automate the process of analyzing documents and extracting data at scale with high accuracy.
Azure Form Recognizer provides numerous pre-built models for analyzing common documents, such as invoices, receipts, and business cards, while you can also build custom models to analyze documents specific to your business.
In this article, we will be focusing on analyzing common sales receipts using a pre-trained receipt model.
To use the Azure Form Recognizer service, you can either create a Form Recognizer resource or a Cognitive Services resource.
In this exercise, you will create a single Form Recognizer resource. If you plan to use Form Recognizer along with other cognitive services, such as Computer Vision, you can create a Cognitive Services resource, or else you can create a Computer Vision resource.
Sign in to Azure Portal and select Create a resource.
Search for Form Recognizer and then click Create.
Create a Form Recognizer resource with the following settings:
Select Review + Create and then select the Create button and wait for the deployment to complete.
Once the resource has been deployed, select Go to resource.
Navigate to the Keys and Endpoint page. Save the Key 1 and the Endpoint. You’ll need these values to connect your Python application to the Form Recognizer API.
To analyze documents with Form Recognizer, you’ll need to install the Form Recognizer client library for Python. You can install the latest version of the client library using pip
:
|
|
Create a configuration file (.env
) and save the Azure’s key and endpoint you copied in the previous step.
Create a new Python file (form-recognizer.py) and import the following libraries:
|
|
Add the following code to load the key and endpoint from the configuration file.
|
|
To interact with the Form Recognizer resource, create a FormRecognizerClient
. Using the methods of the FormRecognizerClient
, you will be able to recognize common fields from receipts.
The following code creates a FormRecognizerClient
using the key
and endpoint
variables you defined above.
|
|
In this article, we will be focusing on analyzing and extracting common fields and semantic values from sales receipts.
Insert the following code after the form recognizer client creation to analyze a receipt from a URL. This code calls the begin_recognize_receipts_from_url
method to extract values from the receipt and then retrieves the result. The input parameter of the above-mentioned method must be the URL of the receipt to be analyzed.
|
|
begin_recognize_receipts
method of the FormRecognizerClient
.Add the following code to print general information about the given receipt, such as the receipt type and the transaction date.
|
|
The following code displays information about the items (such as item name, price, and quantity along with their confidence score) recognized by the pre-trained receipt model.
|
|
Use the following code to print the total tax and the transaction total and subtotal along with the confidence score.
|
|
In this article, you learned how to analyze sales receipts using the Form Recognizer service and the client library for Python. Here are some additional resources from Microsoft Learn:
If you have finished learning, you can delete the resource group from your Azure subscription:
In this article, you will build a document processing solution using Form Recognizer, Logic Apps, and Azure OpenAI.
In this article, you will build an end-to-end document processing solution using Form Recognizer, Logic Apps, and Power BI.
The session “Automate Document Processing with Azure Form Recognizer” presented at EMEA Cloud and Modern Workplace Summit 2023.
In this article, you will train a custom extraction model in Azure Form Recognizer for processing customer reviews.