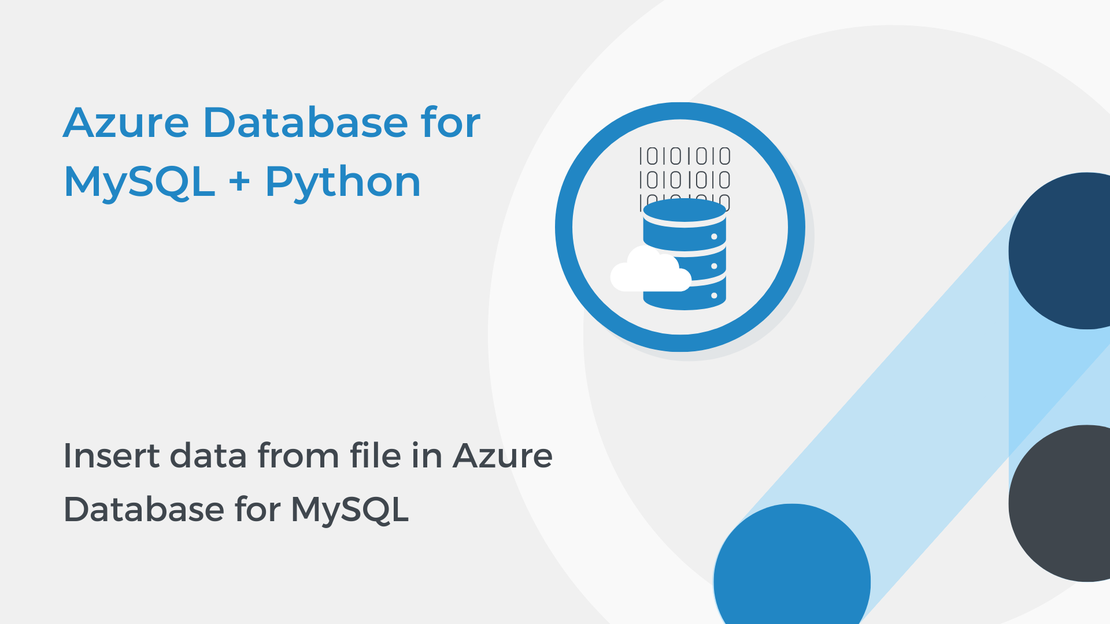
Insert data from file in Azure Database for MySQL
In this tutorial, you will use SQL statements to manipulate data in Azure Database for MySQL and learn how to open files in Python.
This tutorial will show you how to use Python to connect to an Azure Database for MySQL. To complete the exercise, you will need to install the following tools:
If you need help with installing these tools, follow the instructions in the Set up your Python beginner development environment with Visual Studio Code Microsoft Learn Module.
Open the command prompt and run the following command to install the MySQL connector for Python.
|
|
Open Visual Studio Code and create a new Python file. Add the following command and select Run.
|
|
If MySQL Connector is installed successfully, the above code will be executed with no errors.
The next step is to collect the information that you need to connect to the Azure Database for MySQL. You need the server name, the database name, and the login credentials (username and password).
Sign in to Azure Portal, expand the left navigation panel and select All resources.
Then, select the Database that you created in the part 1.
In the Overview tab, you can see the Server name and Server admin login name.
Open Visual Studio Code and create a new Python file named db1.py. Add the following code to connect to your database:
|
|
where:
username
is the admin login name,server
refers to the server name,password
is the password of the admin anddemodb
is the name of the database that you created in part 1.Now you are ready to start working with MySQL in Python. In the following tutorials, you will use Pyhton and SQL statements to create tables, manipulate and query data in the database.
In this tutorial, you will use SQL statements to manipulate data in Azure Database for MySQL and learn how to open files in Python.
This tutorial will show you how to use Python to create tables and manipulate data in Azure Database for MySQL.
In this tutorial, you will learn how to create an Azure Database for MySQL single server, create a new database and configure firewall rules.
This tutorial will show you how to manipulate and query data in an Azure SQL database using Python and Jupyter Notebooks.