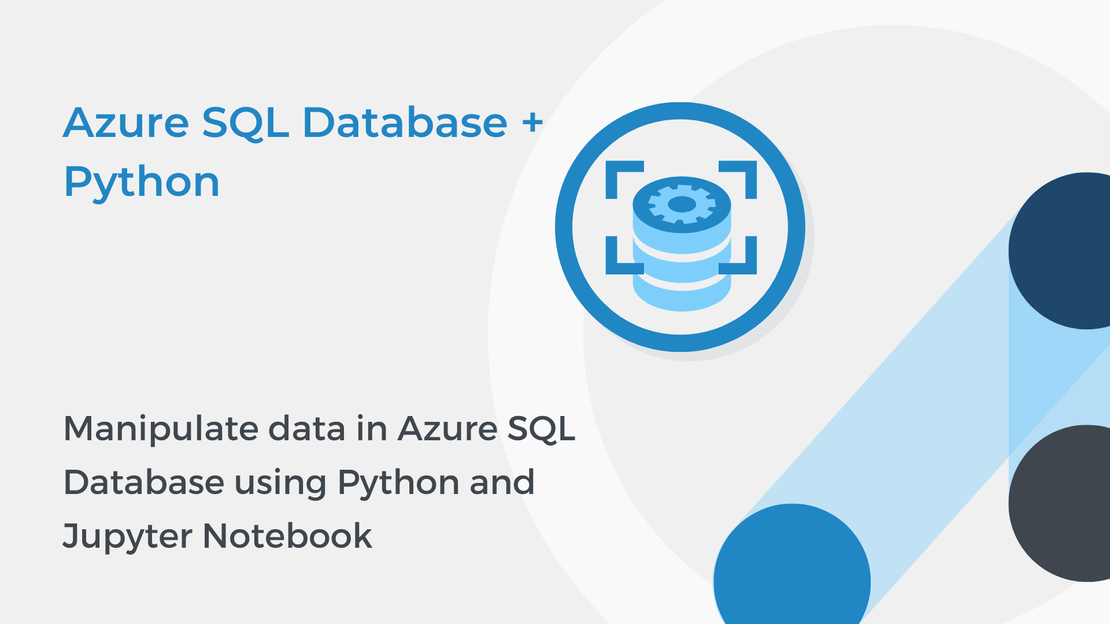
Manipulate data in Azure SQL Database using Python and Jupyter Notebook
This tutorial will show you how to manipulate and query data in an Azure SQL database using Python and Jupyter Notebooks.
In this tutorial, you will learn how to use Python and Jupyter Notebooks to connect to an Azure SQL Database. To complete the exercise, you will need to install:
In this exercise, you will use the pyodbc library to connect to your Azure SQL database and execute SQL queries.
Install the Microsoft ODBC Driver for SQL Server on Windows.
Open the command prompt and run the following command to install the pyodbc library.
|
|
Open the command prompt and run the following command to install the Jupyter Notebook.
|
|
Then, open Visual Studio Code. Go to View > Extensions and search for Jupyter.
Install the Jupyter Extension for Visual Studio Code.
The next step is to collect the information that you need to connect to the Azure SQL Database. You need the server name, the database name, and the login credentials (username and password).
Sign in to Azure Portal, expand the left navigation panel and select All resources.
Select the SQL Database that you have created in the part 1.
In the Overview tab, you can see the fully qualified server name.
Open Visual Studio Code and create a new Jupyter file named connect-sql.ipynb. In the first cell, add the following code to connect to your sql database using Python:
|
|
where:
server
is the server name,database
refers to the name of the database that you created in part 1,username
is the admin login name, andpassword
is the password of the admin.Now you are ready to start working with Azure SQL databases in Python. In the following tutorials, you will learn how to insert a Python dataframe into a SQL table and manipulate data using Python and SQL statements in Jupyter Notebooks.
This tutorial will show you how to manipulate and query data in an Azure SQL database using Python and Jupyter Notebooks.
In this tutorial, you will learn how to use Python and Jupyter Notebooks to insert a dataframe into an Azure SQL table.
The session “Azure SQL Database: Use Python to manipulate data” at Global Azure Greece 2021 by Foteini Savvidou.
In this tutorial, you will learn how to create an Azure SQL Database and configure firewall rules to enable connectivity.