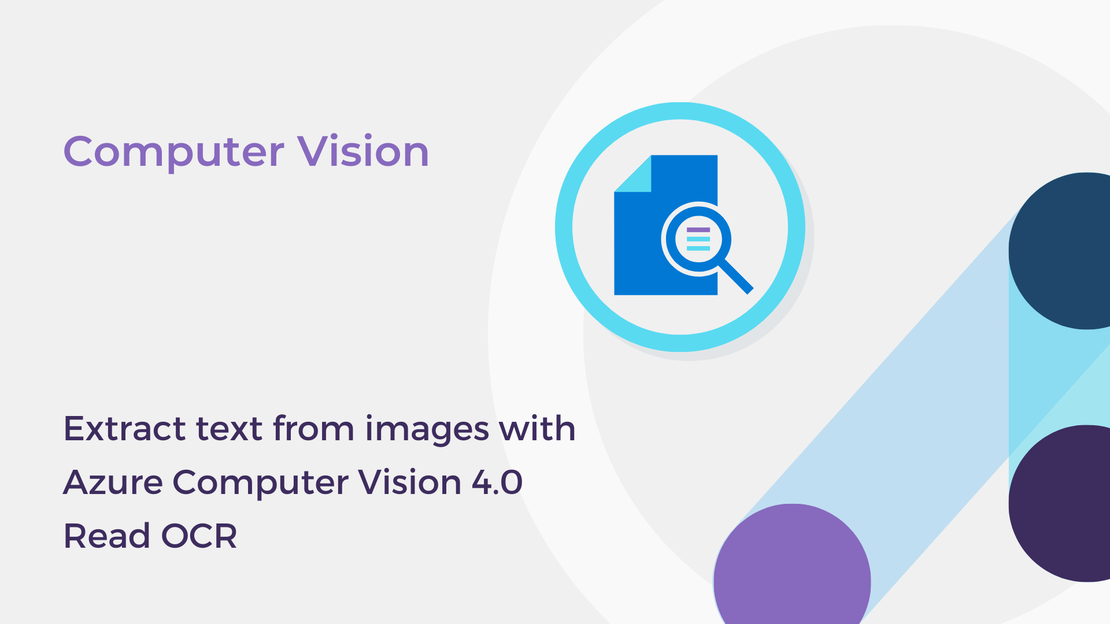
Extract text from images with Azure Computer Vision 4.0 Read OCR
This post will take you through the newest Read OCR API of Azure Computer Vision, which is used for extracting text from images.
Welcome to the new learning series focused on Azure Cognitive Services and Python! In the “Digitize and translate your notes with Azure Cognitive Services and Python” series, you will explore the built-in capabilities of Azure Computer Vision for optical character recognition and the Azure Translator service and build a simple AI web app using Flask.
In the first article, we will explore the pre-trained models of the Azure Computer Vision service for optical character recognition. We will build a simple Python script that turns your handwritten notes into digital documents. You will learn how to:
To complete the exercise, you will need to install:
The Computer Vision service provides pre-built, advanced algorithms that process and analyze images and extract text from photos and documents (Optical Character Recognition, OCR). The READ API uses the latest optical character recognition models and works asynchronously. This means that the READ operation requires a three-step process:
Study the following sketch note to learn more about Optical Character Recognition with the Azure Computer Vision READ API.
You can find more information and how-to-guides about Computer Vision and Optical Character Recognition on Microsoft Learn and Microsoft Docs.
To use the Computer Vision service, you can either create a Computer Vision resource or a Cognitive Services resource. If you plan to use Computer Vision along with other cognitive services, such as Text Analytics, you can create a Cognitive Services resource, or else you can create a Computer Vision resource.
In this exercise, you will create a single Cognitive Services resource to simplify development.
Sign in to Azure Portal and select Create a resource.
Search for Cognitive Services and then click Create.
Create a Cognitive Services resource with the following settings:
Select the required checkboxes and create the resource. Wait for deployment to complete.
Once the resource has been deployed, select Go to resource and view the Keys and Endpoint page. Save the Key 1 and the Endpoint. You will need the key and the endpoint to connect from client applications.
Install the Azure Cognitive Services Computer Vision SDK for Python package with pip
:
|
|
Create a new Python script, for example ocr-demo.py and open it in Visual Studio Code or in your preferred editor.
Want to view the whole code at once? You can find it on GitHub.
Import the following libraries.
|
|
Then, create variables for your Computer Vision resource. Replace YOUR_KEY
with Key 1 and YOUR_ENDPOINT
with your Endpoint.
|
|
Authenticate the client. Create a ComputerVisionClient
object with your key and endpoint.
|
|
First download the images used in the following examples from my GitHub repository.
Add the following code, which submits a local image to the Computer Vision READ API, retrieves and prints the extracted text.
|
|
In this article, you learned how to use Azure Computer Vision READ API to extract text from photos. For more information about using the Azure Cognitive Services Computer Vision SDK for Python package, see the computervision Package documentation.
In the next article, you will learn how to translate text and documents between languages in near real time using the Azure Translator service.
Check out the other parts of the “Digitize and translate your notes with Azure Cognitive Services and Python” series:
If you have finished learning, you can delete the resource group from your Azure subscription:
In the Azure Portal, select Resource groups on the right menu and then select the resource group that you have created.
Click Delete resource group.
This post will take you through the newest Read OCR API of Azure Computer Vision, which is used for extracting text from images.
In this article, you will use the Azure Computer Vision READ API to convert your handwritten notes into digital documents.
In this blog post, you will build a website using Flask and Azure Cognitive Services to extract and translate text from notes.
In this post, you will explore the latest features of Azure Computer Vision and create a basic image analysis app.