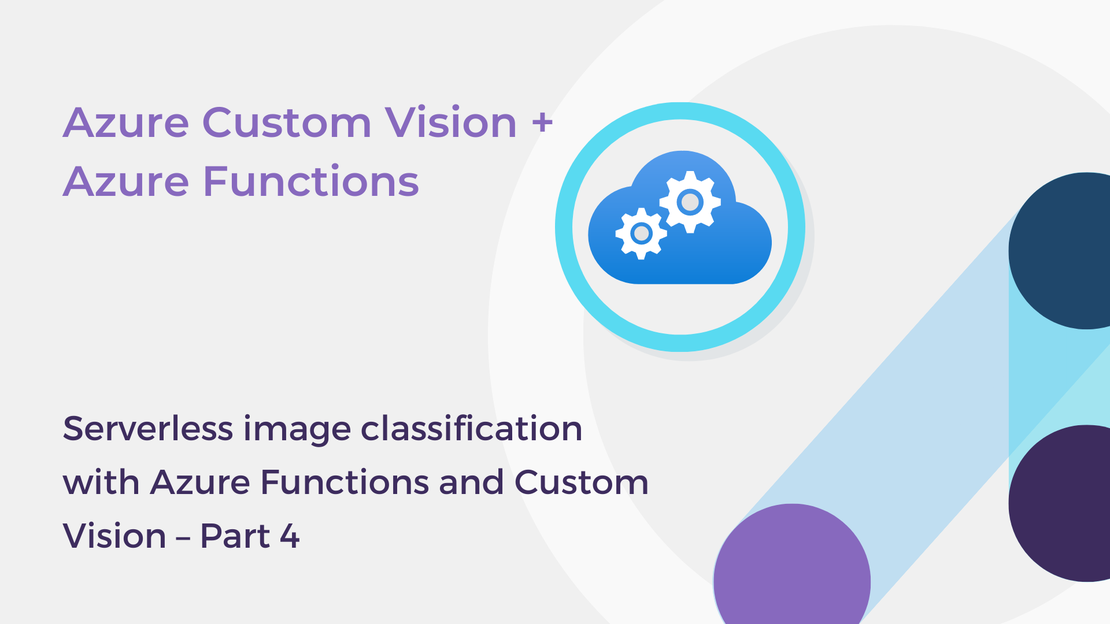
Serverless image classification with Azure Functions and Custom Vision – Part 4
In this article, you will deploy a function project to Azure using Visual Studio Code to create a serverless HTTP API.
Azure Custom Vision Service lets you export your image classification and object detection models to run locally on a device. For example, you can utilize the exported models in mobile applications or run a computer vision model on a microcontroller. You can export a Custom Vision model in numerous formats, including TensorFlow, TensorFlow Lite, TensorFlow.js, ONNX, and Docker containers.
In a previous post, you learned how to train an image classification model using the Python SDK. In this article, you will export the model to a TensorFlow Lite file using the Python client library to fully automate the process of retraining and updating a model.
To complete the exercise, you will need:
Install the Custom Vision client library for Python with pip
:
|
|
Create a configuration file (.env
) and save the key and the endpoint of your training resource and the id of your project and the trained iteration you wish to download.
Create a new Python file (export_model.py) and import the following libraries:
|
|
Add the following code to load the values from the configuration file.
|
|
Use the following code to create a CustomVisionTrainingClient
object. You will use the trainer object to export your model in one of the available formats.
|
|
Add the following code to export the trained iteration to a TensorFlow Lite file and download the exported model. For more information about the export_iteration
method, see the Custom Vision SDK for Python documentation.
|
|
export_iteration
method again. Instead, use the get_exports
method to download the existing exported model.If you have already exported the current iteration to a TensorFlow Lite file, use the following code to download the previously exported model.
|
|
In this article, you learned how to export a Custom Vision trained iteration using the client library for Python. If you are interested in integrating your exported model into an application, you may check out the following resources:
In this article, you will deploy a function project to Azure using Visual Studio Code to create a serverless HTTP API.
In this article, you will create a Python Azure Function with HTTP trigger to consume a TensorFlow machine learning model.
In this article, you will learn how to export a Custom Vision model in TensorFlow format for use with Python apps.
This article will show you how to export your classifier using the Custom Vision SDK for Python and use the model to classify images.