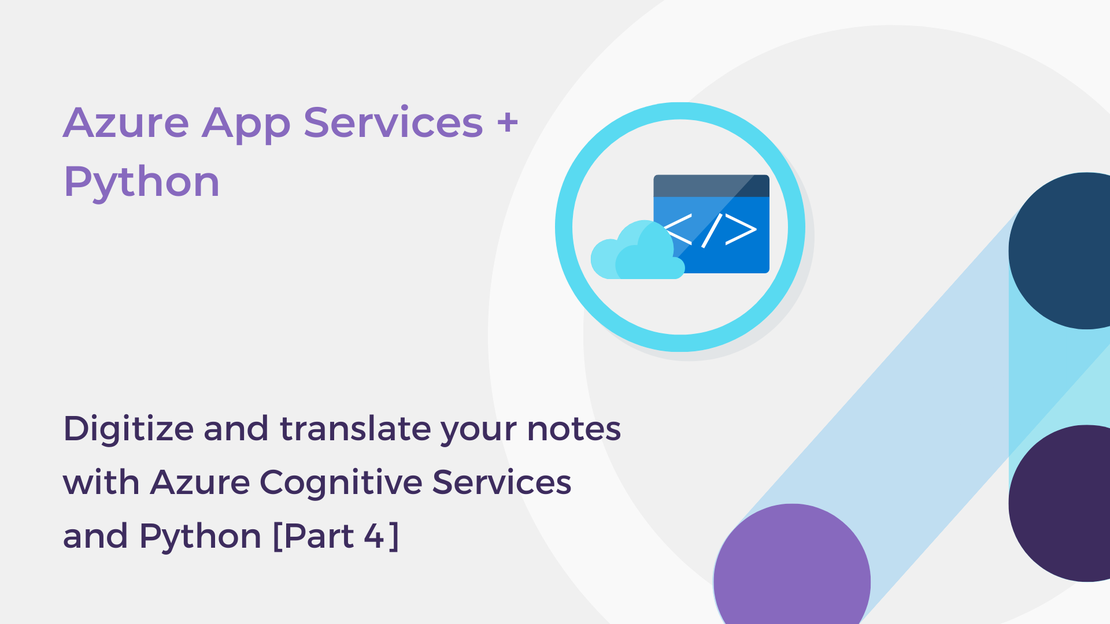
Deploy a Flask AI web app to Azure App Service
In this blog post, you will deploy an AI Flask application app to Azure App Service using Visual Studio Code.
Translator is a cloud-based service that is part of the Azure Cognitive Services and enables you to perform language translation and other language-related operations.
In this article, you will explore the Azure Translator service. You will:
To complete the exercise, you will need to install:
You will also need an Azure subscription. If you don’t have one, you can sign up for an Azure free account.
Study the following sketch note to learn more about the capabilities of the Translator service.
You can find more information and how-to-guides about Translator on Microsoft Learn and Microsoft Docs.
To use the Azure Translator service, you can either create a Translator resource or a Cognitive Services resource. If you plan to use Azure Translator along with other cognitive services, such as Azure Computer Vision, you can create a Cognitive Services resource, or else you can create a Translator resource.
In this exercise, you will create a Translator resource.
Sign in to Azure Portal and select Create a resource.
Search for Translator and then click Create.
Create a Translator resource with the following settings:
Once the deployment is complete, select Go to resource and view the Keys and Endpoint page. Save the Key 1 and the Endpoint for Text Translation. You will need the key and the endpoint to connect from client applications.
Create a configuration file (.env
) and add the authentication key and the region of your Translator resource.
Create a new Python file (translator-demo.py) and import the following libraries.
|
|
Add the following code to load the Translator key from the configuration file and specify the endpoint.
|
|
Define the detect_language
function which automatically detects the language of the source text and returns the language code.
|
|
Add the following code and run the script:
|
|
Define the translate
function which translates the given text from the source_language
into the target_language
and returns the translated text.
|
|
Add the following code and run the script:
|
|
It is also possible to translate the given text into two or more languages. Modify the translate
function as follows:
|
|
Add the following code and run the script:
|
|
In this article, you explored the Azure Translator and build a Python script to detect the language of a given text and translate text from the source language into one or more target languages. For more information about using the Translator service, see the Translator documentation.
If you have finished learning, you can delete the resource group from your Azure subscription:
In this blog post, you will deploy an AI Flask application app to Azure App Service using Visual Studio Code.
In this blog post, you will build a website using Flask and Azure Cognitive Services to extract and translate text from notes.
In this article, you will build a simple Python app that translates text using the Azure Translator service.
Learning resources for the Azure Computer Vision service presented at the community After Party for Microsoft Build 2023 that I organized.