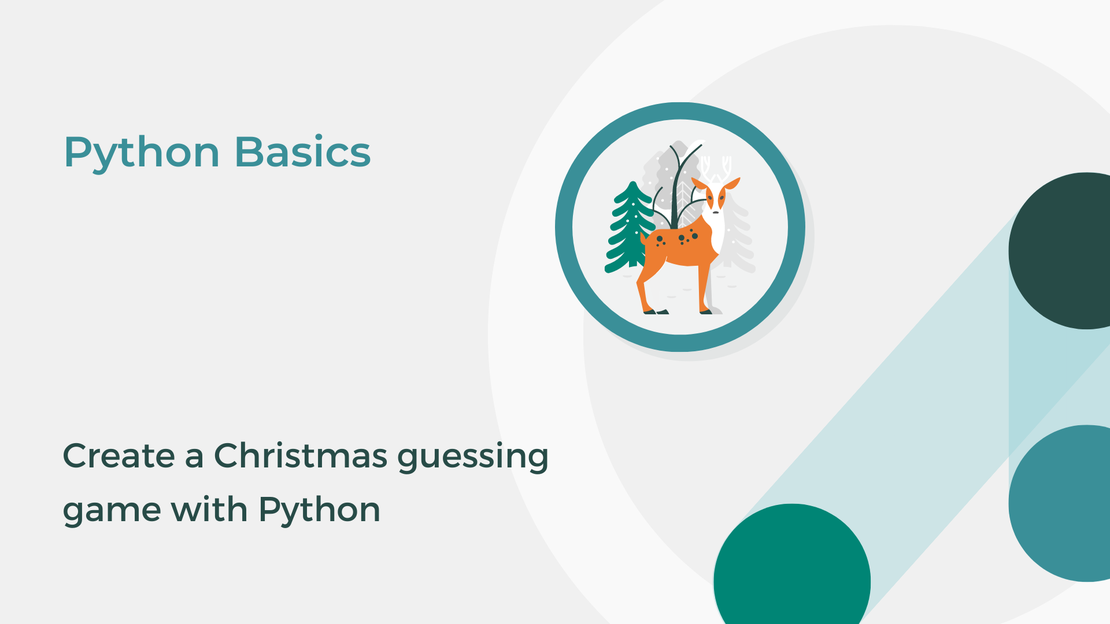
Create a Christmas guessing game with Python
This tutorial will teach you how to build a Christmas “Guess the reindeer” game using Python and Visual Studio Code.
In this tutorial, you will learn how to build the classic Tic Tac Toe game using Python and Visual Studio Code.
Project goal | Get started with Python by creating a player vs computer Tic Tac Toe game |
---|---|
What you’ll learn | Functions, input() and randrange() functions, while and for loops, if-statement |
Tools you’ll need | Python 3, Visual Studio Code and Python extension for Visual Studio Code |
Time needed to complete | 60 minutes |
To complete the exercise, you will need to install:
If you need help with installing these tools, follow the instructions in the Microsoft Learn Module Set up your Python beginner development environment with Visual Studio Code.
Open Visual Studio Code and create a new file named tic-tac-toe.py.
You are going to create the board of the game. Firstly, define the main()
function, which will contain all the game’s code.
|
|
Inside the main()
function, create a two-dimensional list that represents the board of the game.
|
|
Then create the function print_board()
that takes as argument the board of the game and prints it. The rows and the columns of the board are numbered from 1 to 3. We will use the print_board()
function to print the board in every round.
|
|
The game has 2 players:
Create a variable named turn
to store the number of the current player. Player “X” plays first.
|
|
The game ends when the board is full. Therefore, use a while
loop with a condition not is_board_full(board)
to simulate the game.
|
|
where the is_board_full()
function takes as argument the board of the game and checks if the board has empty squares. If the board contains empty squares, the function returns False
.
|
|
while
loop you can repeat a set of instructions as long as a condition is true. You can also use the else
statement to run code when the condition becomes false.Use the else
keyword to print the board of the game and the message “It’s a tie!” at the end of the game.
|
|
In the next sections, you will learn how to check if a player won and end the game (the while
loop) using the break
statement.
Inside the while
loop, the flow of the game will look like this:
print_board()
function to print the board of the game.turn
variable is 0
, the classic player plays.turn
variable is 1
, computer plays.
|
|
Define the function play()
with the board
as argument. This function takes as input from the player the row and the column number and check if their move is valid.
input()
function to get new input from the player.input()
function. The input()
function has an optional parameter, known as prompt, which is a string that will be printed before the input.
|
|
In the main()
function, call the play()
function to get the row and column numbers and then update the board of the game.
|
|
Define the function play_random()
with the board
as argument. This function finds all the possible moves (the squares of the board that are empty) and stores the row and the column numbers of the valid squares in the list possible_moves
. Then it randomly selects one of the valid squares and returns the row and column numbers of the selected square.
Use the command:
|
|
to generate a random number in the range of 0
(included) to len(possible_moves)
(not included), where len(possible_moves)
is the length of the list possible_moves
.
Then use this number to select the corresponding list item:
|
|
and return it.
|
|
In short, the randrange()
function returns a randomly selected number from a specified range. We can use two parameters to specify the lower and higher limit. For example, randrange(1, 10)
will return a number between 1
(included) and 10
(not included) and randrange(10)
will return a number between 0
(included) and 10
(not included).
In order to use the randrange()
function you should add the following lines of code at the beginning of your program:
|
|
In the main()
function, call the play_random()
function to get the random row and column numbers and then update the board of the game.
|
|
The last step of the game is to find the player that won. A player wins, if they succeed in placing three of their marks in a diagonal, horizontal, or vertical row.
Firstly, define the function check_winner()
, which takes as argument the board of the game and uses the functions check_row()
, check_column()
, check_diagonals()
to determine if a player won.
|
|
The check_row()
function takes as arguments the board of the game and the number of the board’s row and returns True
if a player has placed three of their marks in the selected row.
|
|
The check_column()
function takes as arguments the board of the game and the number of the board’s column and returns True
if a player has placed three of their marks in the selected column.
|
|
Lastly, the check_diagonals()
function takes as argument the board of the game and returns True
if a player has placed three of their marks in a diagonal.
|
|
In the main()
function, inside the while
loop, use the check_winner()
function to check if a player wins. If a player wins, print the board of the game and an appropriate message and then use the break
statement to end the game.
|
|
Congratulations! You’ve made it! You have developed a Tic Tac Toe game in Python.
|
|
This tutorial will teach you how to build a Christmas “Guess the reindeer” game using Python and Visual Studio Code.
Explore how I use GitHub Copilot in my daily development workflow and discover features you may not have come across yet.
Explore how to automate the build and deployment process for a visual novel game using GitHub Actions and Azure Static Web Apps.
Explore how I built an educational game using the Ren'Py visual novel engine and AI tools like GitHub Copilot and DALL-E 3 to speed up development.