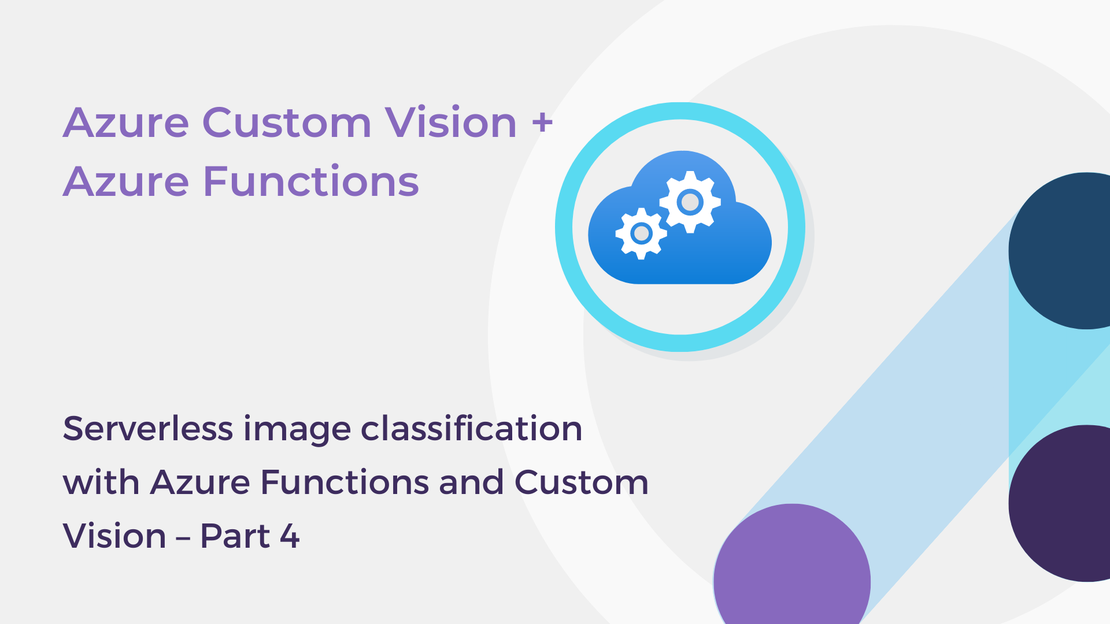
Serverless image classification with Azure Functions and Custom Vision – Part 4
In this article, you will deploy a function project to Azure using Visual Studio Code to create a serverless HTTP API.
One important research area in precision agriculture is the detection of diseases from images of plant leaves. How about using the Azure Custom Vision service to help farmers identify plant diseases from images?
In this article, you will build and deploy a custom vision model for identifying tomato leaf diseases. You will learn how to:
To complete the exercise, you will need:
You will also need to install Python 3 and Visual Studio Code or another code editor.
Azure Custom Vision is an Azure Cognitive Services service that lets you build and deploy your own image classification and object detection models. You can train your models using either the Custom Vision web portal or the Custom Vision SDKs.
Do you want to learn more about Azure Custom Vision? You can read my previous articles about creating a Custom Vision model for flower classification and an object detection model for grocery checkout.
Images of tomato leaf diseases were taken from the Plant Leaf Diseases database by Arun Pandian et al. This dataset is composed of 61,486 images, which are divided into 39 classes of plant leaf diseases. To build and train our Custom Vision model, we will only consider the following classes:
We will use 60 images per class for the first training iteration. You can download the dataset employed in this project from the Mendeley Data website.
Once you have created a Custom Vision resource in the Azure portal, you will need the key and endpoint of your training and prediction resources to connect your Python application to Custom Vision. You can find these values both in the Azure portal and the Custom Vision web portal.
Navigate to customvision.ai and sign in.
Click the settings icon (⚙) at the top toolbar.
Expand your prediction resource and save the Key, the Endpoint, and the Prediction resource ID.
Expand the training resource and save the Key and the Endpoint.
To create an image classification project with Custom Vision for Python, you’ll need to install the Custom Vision client library. Install the Azure Cognitive Services Custom Vision SDK for Python package with pip
:
|
|
Create a configuration file (.env
) and save the Azure’s keys and endpoints you copied in the previous step.
Create a new Python file (app.py) and import the following libraries:
|
|
Add the following code to load the keys and endpoints from the configuration file.
|
|
Use the following code to create a CustomVisionTrainingClient
and CustomVisionPredictionClient
object. You will use the trainer
object to create a new Custom Vision project and train an image classification model and the predictor
object to make a prediction using the published endpoint.
|
|
To create a new Custom Vision project, you use the create_project
method of the trainer
object. By default, the domain of the new project is set to General. If you want to export your model to run locally on a device, you should select one of the compact domains.
Add the following code to create a new “Multiclass” classification project in the General (compact) domain.
|
|
The images that we will use to build our model are grouped into 10 classes (tags). Insert the following code after the project creation. This code specifies the description of the tags and the names of the folders with the training images of each class and adds classification tags to the project using the create_tag
method.
|
|
The following code uploads the training images and their corresponding tags. In each batch, it uploads 60 images of the same tag.
|
|
Use the train_project
method of the trainer
object to train your model using all the images in the training set.
|
|
The Custom Vision service calculates three performance metrics:
The following code displays performance information for the latest training iteration using a threshold value equal to 50%. You can also use the get_iteration_performance
method to get the standard deviation of the above-mentioned performance values.
|
|
Add the following code, which publishes the current iteration. Once the iteration is published, you can use the prediction endpoint to make predictions.
|
|
Use the classify_image
method of the predictor
object to send a new image for analysis and retrieve the prediction result. Add the following code to the end of the program and run the application.
|
|
If the application ran successfully, navigate to the Custom Vision website to see the newly created project.
In this article, you learned how to use the Azure Custom Vision SDK for Python to build and train an image classification model. You may check out the following article on Microsoft Docs about how to retrain the model in order to make it more accurate: How to improve your Custom Vision model – Microsoft Docs.
If you want to delete this project, navigate to the Custom Vision project gallery page and select the trash icon under the project.
If you have finished learning, you can delete the resource group from your Azure subscription:
References:
[1] J, ARUN PANDIAN; GOPAL, GEETHARAMANI (2019), “Data for: Identification of Plant Leaf Diseases Using a 9-layer Deep Convolutional Neural Network”, Mendeley Data, V1, doi: 10.17632/tywbtsjrjv.1
[2] Geetharamani G., Pandian A., Identification of plant leaf diseases using a nine-layer deep convolutional neural network, Computers and Electrical Engineering, 2019, doi: 10.1016/j.compeleceng.2019.04.011
In this article, you will deploy a function project to Azure using Visual Studio Code to create a serverless HTTP API.
In this article, you will create a Python Azure Function with HTTP trigger to consume a TensorFlow machine learning model.
This article will show you how to export your classifier using the Custom Vision SDK for Python and use the model to classify images.
In the first article of this series, you will build and deploy an image classification model using the Custom Vision SDK for Python.