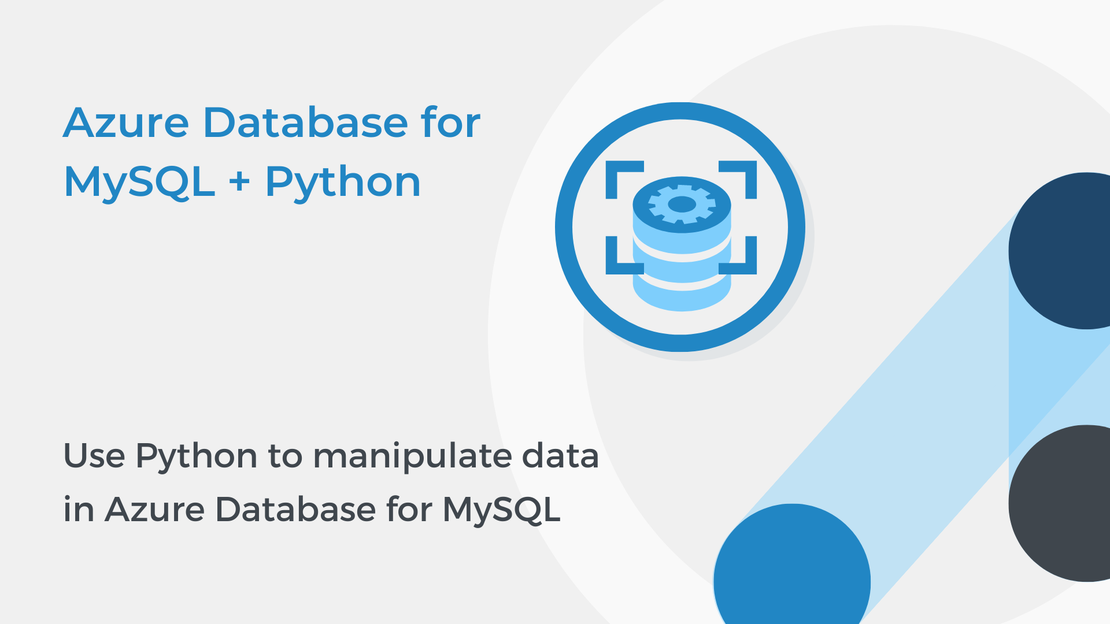
Use Python to manipulate data in Azure Database for MySQL
This tutorial will show you how to use Python to create tables and manipulate data in Azure Database for MySQL.
In this tutorial, you will create a simple database for a library and learn how to open and manage .txt files in Python. You will also use INSERT
, SELECT
, UPDATE
and DELETE
statements to manipulate data in Azure Database for MySQL. To complete the exercise, you will need to install:
If you need help with installing these tools, follow the instructions in the tutorial Connect to Azure Database for MySQL using Python.
You can download the code of this tutorial from my GitHub repository.
Open Visual Studio Code and create a new Python file named db3.py. Copy the code from the previous tutorials to connect to your database and create a cursor object.
The following code creates a table named library
. The table has five columns:
id
column is used to uniquely identify a row (PRIMARY KEY
) and it is an integer (INT
).title
, author
and genre
columns is VARCHAR
which stands for variable length character data.quantity
column specifies the number of the available books.
|
|
A file Input/Output (IO) requires three steps:
open()
function.close()
function.Assume that we have the books.txt file located in the same folder as the Python program. You can use a with
statement and the open()
function to open the file.
|
|
Then use a for
loop to read the file line-by-line, the strip()
function to remove the newline character at the end of each line and the split()
function to split the string into a list. To load data, use the INSERT
SQL statement. As you learned in the previous tutorial, in an INSERT
statement, you specify a table and columns in an INTO
clause, and a list of values to be stored in these columns in a VALUES
clause. You can use a %s
marker to specify the parameters in the SQL statement and add a tuple containing the values.
Use the following code to open the file and insert data into the table.
|
|
In the previous tutorial, you learned that you can read the data by using a SELECT
statement. The following code selects all the rows of the table and reads the data using the fetchall()
method.
|
|
You can use the .rowcount
property to print the number of rows returned by a SELECT
statement.
|
|
Then you can use a simple for
loop to iterate over the rows and print the data.
|
|
The next example shows how to update an existing record using an UPDATE
SQL statement. It reduces by 1 the quantity
of the book with id
equal to 18
. All other rows are left unchanged.
|
|
The following code increases by 1 the quantity
of the books with id
equal to 10
or 22
. All other rows are left unchanged.
|
|
You can also use the .rowcount
property to print the numbers of rows affected by the UPDATE
statement.
|
|
Use the following code to commit the changes and close the cursor and the connection.
|
|
This tutorial will show you how to use Python to create tables and manipulate data in Azure Database for MySQL.
In this tutorial, you will learn how to connect to your Azure Database for MySQL using Python and Visual Studio Code.
In this tutorial, you will learn how to create an Azure Database for MySQL single server, create a new database and configure firewall rules.
This tutorial will show you how to manipulate and query data in an Azure SQL database using Python and Jupyter Notebooks.