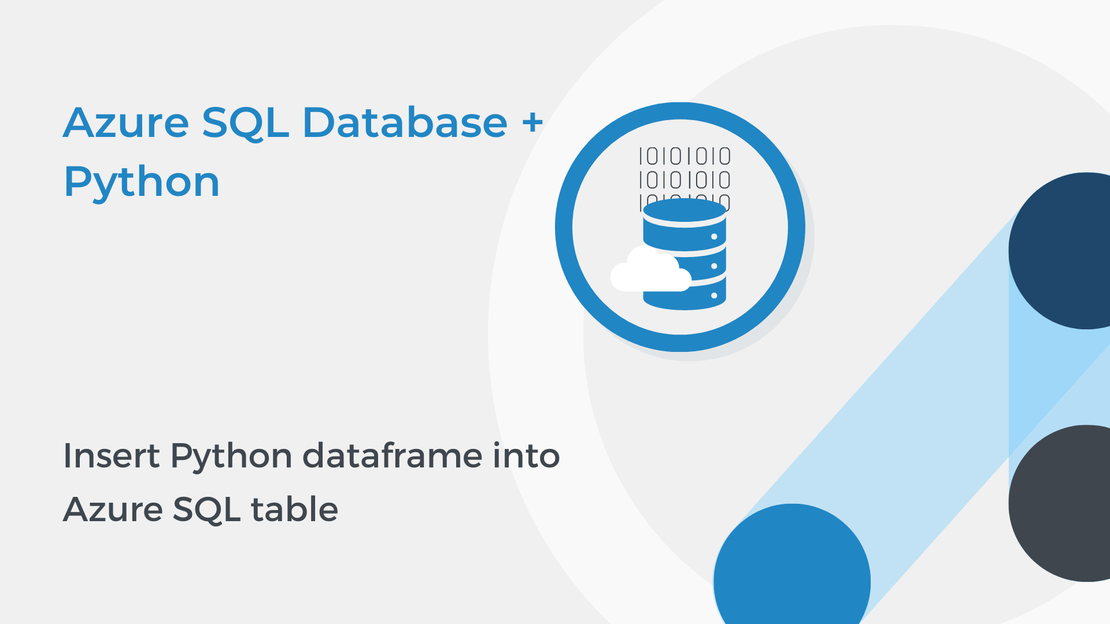
Insert Python dataframe into Azure SQL table
In this tutorial, you will learn how to use Python and Jupyter Notebooks to insert a dataframe into an Azure SQL table.
This tutorial will show you how to manipulate and query data in an Azure SQL database using Python and Jupyter Notebooks in Visual Studio Code. To complete the exercise, you will need to install:
pip
.If you need help with installing these tools, follow the instructions in the tutorial Connect to Azure SQL Database using Python and Jupyter Notebook.
You can download the code from my GitHub repository.
Open Visual Studio Code and create a new Jupyter file named manipulate-data-sql.ipynb. Copy the code from the previous tutorial to connect to your Azure SQL database and create a cursor
object.
The following code adds a new movie in the table using the INSERT
statement.
INSERT
statement, you specify a table and columns in an INTO
clause, and a list of values to be stored in these columns in a VALUES
clause.
|
|
It is important to use the commit()
method after every transaction that modifies data.
|
|
You can read data by using a SELECT
statement. Use the following code to select the id, the title and the director of all the movies in the table and read the data using the fetchall()
method.
|
|
Then you can use a simple for
loop to iterate over the rows and print the data.
|
|
In SQL we use the UPDATE
statement to modify existing records in a table. The next example shows how to update the genre of a movie.
|
|
WHERE
clause in the UPDATE
statement. The WHERE
clause specifies that only the movie “The Theory of Everything” should be updated. If you omit the WHERE
clause, all the records in the table will be updated!Then you can use the .rowcount
property to print the number of rows updated by the previous UPDATE
statement.
|
|
In the next cell of your notebook, you can use the following code to print the genre of the movie “The Theory of Everything” to verify that you have successfully updated the genre.
|
|
You can use a DELETE
statement to delete existing records in a table. The following code deletes all the horror movies and prints the number of the movies that have been removed from the table.
|
|
WHERE
clause in the above DELETE
statement. If you omit the WHERE
clause, all records in a table will be deleted!Now that you have learned the basics, you can perform complex queries. Select all the action movies from the movies
table that released between 2009 and 2019, sorted ascending by the released_year
and descending by the gross
.
|
|
MIN
, MAX
, COUNT
, AVG
, SUM
, etc. to perform calculations on a set of values. Aggregate functions return a single value.Using the MAX()
function, find and print the gross of the highest-grossing movie of Martin Scorsese.
|
|
Use the following code to close the cursor and the connection.
|
|
Congratulations! In the “Azure SQL Database and Python” tutorial series, you learned how to create an Azure SQL Database and connect to the database, create tables, manipulate, and query data by using Python and Jupyter Notebooks.
If you are interested in getting started with Azure databases and Python, you can read the tutorial series that I have written about how to manipulate and query data in Azure Database for MySQL by using Python.
In this tutorial, you will learn how to use Python and Jupyter Notebooks to insert a dataframe into an Azure SQL table.
In this tutorial, you will learn how to use Python and Jupyter Notebooks to connect to an Azure SQL Database.
The session “Azure SQL Database: Use Python to manipulate data” at Global Azure Greece 2021 by Foteini Savvidou.
In this tutorial, you will learn how to create an Azure SQL Database and configure firewall rules to enable connectivity.