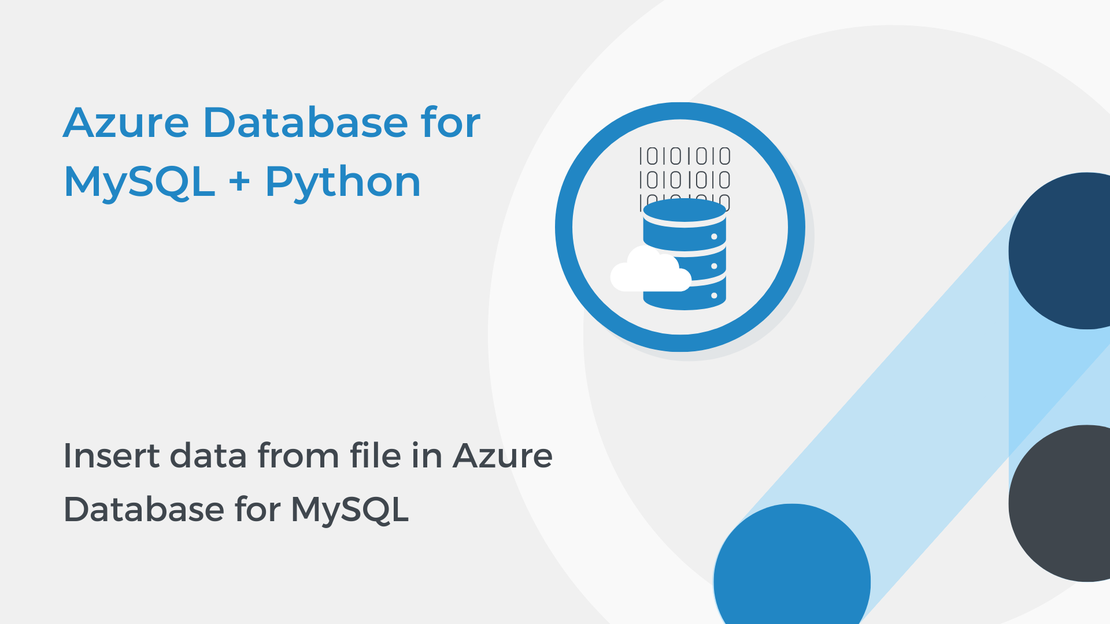
Insert data from file in Azure Database for MySQL
In this tutorial, you will use SQL statements to manipulate data in Azure Database for MySQL and learn how to open files in Python.
This tutorial will show you how to use Python to create tables and manipulate data in Azure Database for MySQL. You will learn how to insert, read, update, and delete data. To complete the exercise, you will need to install:
If you need help with installing these tools, follow the instructions in the tutorial Connect to Azure Database for MySQL using Python.
You can download the code of this tutorial from my GitHub repository.
Open Visual Studio Code and create a new Python file named db2.py. Copy the code from the previous tutorial to connect to your database.
|
|
Cursor objects can interact with the MySQL server and execute operations such as SQL statements. To create a cursor, use the cursor()
method of the conn
object.
|
|
To execute SQL statements you can use the execute()
method of the cursor
object.
The following example creates a new table named books
. The items between the parentheses specify the details of each column. The table has four columns:
The id
column is used to uniquely identify a row (PRIMARY KEY
) and it is an integer (INT
). The AUTO_INCREMENT
keyword allows a unique number to be created automatically when a new record is inserted into the table.
The data type of the title
, author
and genre
columns is VARCHAR
which stands for variable length character data.
|
|
To load data, use the INSERT
SQL statement.
INSERT
statement, you specify a table and columns in an INTO
clause, and a list of values to be stored in these columns in a VALUES
clause.
|
|
It is possible to write the INSERT
statement in a slightly different form. You can use a %s
marker to specify the parameters in the SQL statement and add a tuple containing the values.
|
|
It is important to use the commit()
method after every transaction that modifies data.
|
|
Use the following code to add three more books into the table.
|
|
You can read the data by using a SELECT
statement. The following code selects all the rows of the table and reads the data using the fetchall()
method.
|
|
Then you can use a simple for
loop to iterate over the rows and print the data.
|
|
You can use the executemany()
method to insert multiple rows at once. You specify the parameters in a list and the executemany()
method executes a SQL command against all parameter sequences found in that list.
Run the following code to insert 11 new books into the books
table.
|
|
The next example shows how to update an existing record using an UPDATE
SQL statement. It modifies the genre of the book “Animal Farm”. All other rows are left unchanged.
|
|
You can use a DELETE
statement to delete existing records in a table. The following code removes the book “Hamlet” from the table.
|
|
WHERE
clause specifies the records to be deleted. If you omit the WHERE
clause, all rows in the table will be deleted!In a WHERE
clause, you can use operators such as the OR
operator to specify which records will be deleted. For example, the following statement deletes all the books by J. R. R. Tolkien and Mark Twain.
|
|
Use the following code to close the cursor and the connection.
|
|
In the next tutorial, you will build a simple database for a small library and learn how to insert data from a .txt file and manipulate data in Azure Database for MySQL by using Python.
In this tutorial, you will use SQL statements to manipulate data in Azure Database for MySQL and learn how to open files in Python.
In this tutorial, you will learn how to connect to your Azure Database for MySQL using Python and Visual Studio Code.
In this tutorial, you will learn how to create an Azure Database for MySQL single server, create a new database and configure firewall rules.
This tutorial will show you how to manipulate and query data in an Azure SQL database using Python and Jupyter Notebooks.