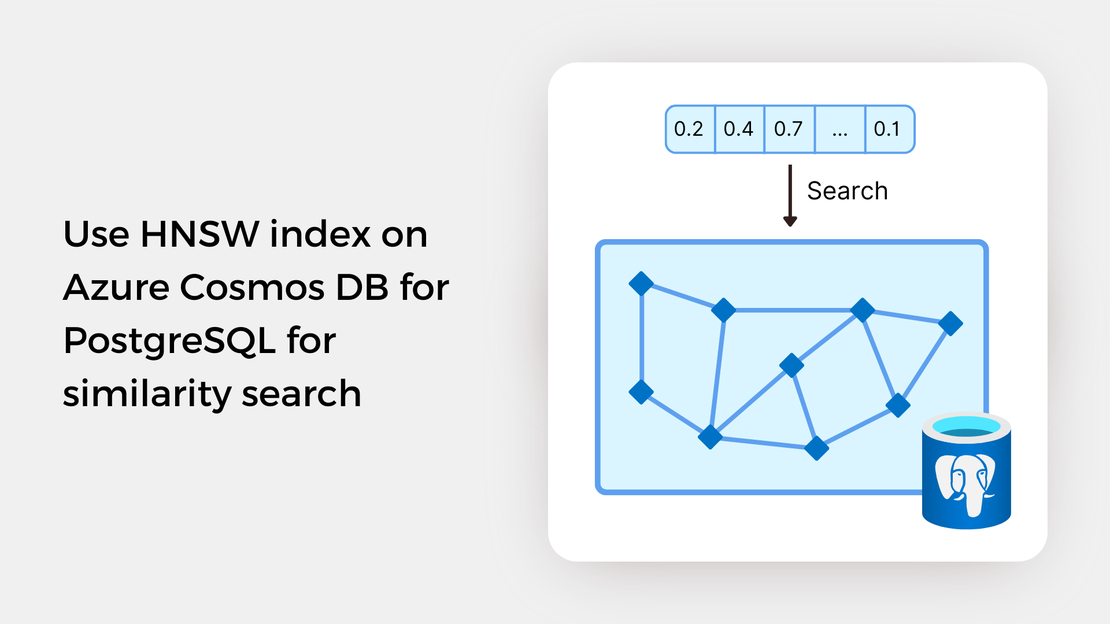
Use HNSW index on Azure Cosmos DB for PostgreSQL for similarity search
Explore vector similarity search using the Hierarchical Navigable Small World (HNSW) index of pgvector on Azure Cosmos DB for PostgreSQL.
This month Microsoft announced the public preview of the Azure AI extension on Azure Database for PostgreSQL Flexible Server which simplifies the integration of various Azure AI Services, including Azure OpenAI and Azure AI Language, with your data. Through the azure_ai
extension, you can generate, store, index, and query embeddings using SQL code.
In this article, you will learn how to:
azure_ai
extension.For a quick introduction to the concepts of vector similarity search and vector embeddings, you can read my post Building a vector similarity search app with Azure AI Vision and PostgreSQL.
To proceed with this tutorial, ensure that you have the following prerequisites installed and configured:
text-embedding-ada-002
deployed: You can request access to the Azure OpenAI service by filling out the application form.Before installing an extension in Azure Database for PostgreSQL Flexible Server, you should add it in the list of the allowed extensions.
azure.etensions
parameter and add the AZURE_AI
and VECTOR
extensions to the allowlist.azure_ai
and the pgvector (vector
) extensions because they are commonly used together.Then, you can install the extension, by connecting to your database and running the CREATE EXTENSION
command from the psql
command prompt:
|
|
The azure_ai
extension includes functions that allow you to call the Azure OpenAI service and generate embeddings within your database. In order to use this functionality, you should first specify the endpoint and the key of your Azure OpenAI resource.
The azure_ai.set_setting()
function lets you set the necessary configuration values for Azure AI services. Execute the following commands from the psql
command prompt to specify the endpoint and the key of your Azure OpenAI resource:
|
|
The code provided illustrates the core features of the Azure AI extension. Assume that you want to create a database to store blog post details, such as titles and abstracts. Your goal is to generate vector embeddings from the text data provided to search for similar articles more effectively.
|
|
In the following sections, we’ll delve into creating a table to store vector embeddings, generating embeddings with Azure OpenAI, and searching for similar vectors.
The pgvector extension introduces a data type called VECTOR
for indicating that a table column holds vector embeddings. When creating the column, it’s essential to specify the dimension of the vectors. In our scenario, the text-embedding-ada-002
generates 1536-dimensional vectors.
Additionally, we want to specify that the vector
column is automatically populated with data upon the insertion of a new record into the table. The vector embeddings are generated by concatenating the title
and abstract
fields and calling the text-embedding-ada-002
model.
|
|
We will insert information (title
and abstract
) about 5 blog posts into the table. Notice that the vector embeddings are created automatically.
|
|
After populating the table with vector data, you can search for blog posts that are most similar to a text prompt. To calculate similarity and retrieve images, you will use SELECT
statements and the built-it vector operators of the PostgreSQL database. To calculate the vector embedding of the search terms, you will use the azure_ai
extension.
The following query computes the negative inner product (<#>
) between the vector of the search_query
and the vectors stored in the table, sorts the results by the calculated distance, and returns the title
and the abstract
of the posts.
|
|
In this post, we explored the basics of the Azure AI extension in Azure Database for PostgreSQL Flexible Server. Here are some resources to learn more about vector similarity search in PostgreSQL:
Explore vector similarity search using the Hierarchical Navigable Small World (HNSW) index of pgvector on Azure Cosmos DB for PostgreSQL.
Explore vector similarity search using the Inverted File with Flat Compression (IVFFlat) index of pgvector on Azure Cosmos DB for PostgreSQL.
Learn how to write SQL queries to search for and identify images that are semantically similar to a reference image or text prompt using pgvector.
My presentation about vector search with Azure AI Vision and Azure Cosmos DB for PostgreSQL at the Azure Cosmos DB Usergroup.